はじめに
本記事は、intel RealSenseを使用して、点群・テキスチャ情報を.ply形式で保存します。
RealSense公式上のサンプルを使用します。
私の学習を兼ねてサンプルコードを少し深堀して書きますので参考にどうぞ。
リンク
リンク
実行環境
本記事の実行環境です
- OS:Windows 10
- intel RealSense D435i
- OpenCV Ver.4.10.0
- Python Ver.3.11
- pyrealsense2 Ver.2.55.1
インストール方法は以下です。
pip install pyrealsense2
サンプルコードおよび.ply形式で保存した点群情報
以下、サンプルコードです。
## License: Apache 2.0. See LICENSE file in root directory.
## Copyright(c) 2017 Intel Corporation. All Rights Reserved.
#####################################################
## Export to PLY ##
#####################################################
# First import the library
import pyrealsense2 as rs
# Declare pointcloud object, for calculating pointclouds and texture mappings
pc = rs.pointcloud()
# We want the points object to be persistent so we can display the last cloud when a frame drops
points = rs.points()
# Declare RealSense pipeline, encapsulating the actual device and sensors
pipe = rs.pipeline()
config = rs.config()
# Enable depth stream
config.enable_stream(rs.stream.depth)
# Start streaming with chosen configuration
pipe.start(config)
# We'll use the colorizer to generate texture for our PLY
# (alternatively, texture can be obtained from color or infrared stream)
colorizer = rs.colorizer()
try:
# Wait for the next set of frames from the camera
frames = pipe.wait_for_frames()
colorized = colorizer.process(frames)
# Create save_to_ply object
ply = rs.save_to_ply("1.ply")
# Set options to the desired values
# In this example we'll generate a textual PLY with normals (mesh is already created by default)
ply.set_option(rs.save_to_ply.option_ply_binary, False)
ply.set_option(rs.save_to_ply.option_ply_normals, True)
print("Saving to 1.ply...")
# Apply the processing block to the frameset which contains the depth frame and the texture
ply.process(colorized)
print("Done")
finally:
pipe.stop()
上記プログラムを実行すると、下記のようなテキスチャ情報が取得できます。
近い側が青、遠くなるにつれて赤が強くなります。
!注意!本サンプルコードでは表示までは実行されません。表示については別記事に記載しましたので参考にしてください。
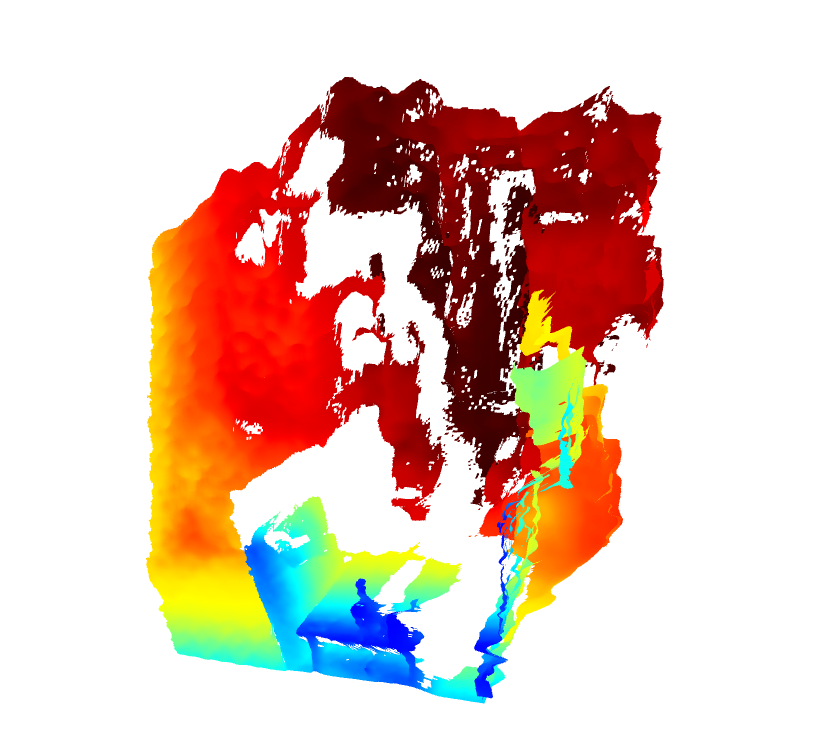
サンプルコードを深堀してみる
ここからは、筆者の学習を兼ねてサンプルコードを深堀します。
# First import the library
import pyrealsense2 as rs
最初に、Intel RealSense SDK 2.0のpython wrapperをrsとしてインポートします。
# Declare pointcloud object, for calculating pointclouds and texture mappings
pc = rs.pointcloud()
# We want the points object to be persistent so we can display the last cloud when a frame drops
points = rs.points()
- pc = rs.pointcloud()
pyrealsense2.pointcloudクラスをpcとして宣言します。このクラスにより深度情報やカラーマップから点群を所得することが可能となります。 - points = rs.points()
pyrealsense2.pointsクラスをpointsとして宣言します。
# Declare RealSense pipeline, encapsulating the actual device and sensors
pipe = rs.pipeline()
config = rs.config()
# Enable depth stream
config.enable_stream(rs.stream.depth)
# Start streaming with chosen configuration
pipe.start(config)
深度情報を所得するストリーミングを準備・開始します。詳細は別記事に記載しました。
# We'll use the colorizer to generate texture for our PLY
# (alternatively, texture can be obtained from color or infrared stream)
colorizer = rs.colorizer()
pyrealsense2.colorizerをcolorizerとして宣言します。
try:
# Wait for the next set of frames from the camera
frames = pipe.wait_for_frames()
colorized = colorizer.process(frames)
- frames = pipe.wait_for_frames()により新しいフレームセットを待ちます(別記事参照)
- colorized = colorizer.process(frames)
公式ドキュメントに詳細な説明はありませんでした。(後日追記)。
pyrealsense2.frame型のcolorizedとしてオブジェクトを生成します。後述する点群などのデータ保存のための準備です。
# Create save_to_ply object
ply = rs.save_to_ply("1.ply")
pyrealsense2.save_to_plyクラスを実行し、plyオブジェクトを生成します。引数として、点群等情報を保存するファイル名をstr型で”1.ply”として指定します。
# Set options to the desired values
# In this example we'll generate a textual PLY with normals (mesh is already created by default)
ply.set_option(rs.save_to_ply.option_ply_binary, False)
ply.set_option(rs.save_to_ply.option_ply_normals, True)
- コメントには”オプションを必要な値に設定する”、”この例では,法線を持つテキストPLYを生成します(メッシュはデフォルトで作成済みです)”と記載されています
- pyrealsense2.save_to_plyクラスのメソッドset_optionにて、保存のためのオプション設定を行います。それぞれのオプション詳細は別記事にまとめました。
- ply.set_option(rs.save_to_ply.option_ply_binary, False)
plyの保存形式は「ASCII」または「バイナリ」の二種類です。このオプション設定ではバイナリ形式を偽で設定、つまりASCII形式での保存を設定していることと同義です。 - ply.set_option(rs.save_to_ply.option_ply_normals, True)
法線(normals)情報の保存を有効にしています。
- ply.set_option(rs.save_to_ply.option_ply_binary, False)
print("Saving to 1.ply...")
# Apply the processing block to the frameset which contains the depth frame and the texture
ply.process(colorized)
print("Done")
コメントの通り、深度フレームとテクスチャを含むフレームセットに処理ブロックを適用します。
フレームセットと引数として、前述したオプション設定などをもとに点群・表面情報を保存します。
finally:
pipe.stop()
最後にストリーミングを終了します。
まとめ
本記事ではintel RealSenseとサンプルコードを使用して、点群・テキスチャ情報を.ply形式で保存する方法を説明しました。
次記事では、保存した.ply形式ファイルの読み込みと点群やテキスチャ情報の表示方法について紹介します。
参考リンク
リンク
リンク